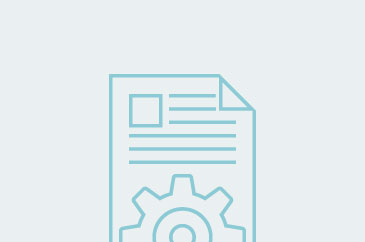
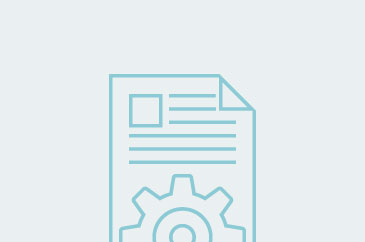
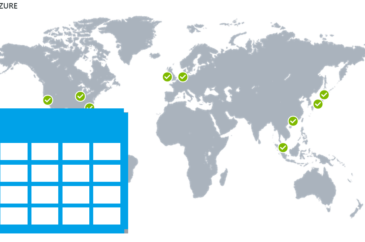
Jeffrey Richter’s Guide to Working with Azure Storage Tables via C# and other .NET Languages
I have been working with Azure Storage Tables for many years now. During this time, I have learned many good practices and have also experienced many bad practices. So, over the past few months, I decided to write a document so I can share my experience with others. I call this document Jeffrey Richter's Guide to Working with Azure Storage Tables via C# and other .NET Languages. You can download my guide from the Wintellect website and you can learn more about Azure Tables by watching my video on the WintellectNOW website. My Guide has been reviewed and is endorsed by Microsoft’s own Azure Storage team. The guide has several purposes:
- Help developers improve their mental model with respect to Azure Tables
- Discuss the good and bad parts of Microsoft's .NET Azure Storage client library
- Show good patterns and practices related to Azure Tables Introduce my own (free) .NET Azure Storage client library which increases programmer productivity. The library offers many features to assist developers working with Azure Storage. For example, it offers blob logging features and a periodic elector which uses blob leases to elect a single VM to perform a periodic task (like backup storage data or to produce a weekly report). For tables, it offers many features including backup/restore, optimistic concurrency, easy filter construction, simple segmented result processing, property replacer/changer, pattern for extensible entity schemas, collection to/from property serializer.
I hope users of Azure Storage find my Guide and its accompanying class library useful.
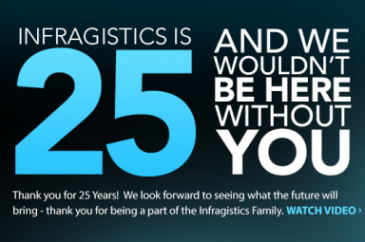
Infragistics Seminar Questions
I did an online session today celebrating the 25th anniversary of our Partner Infragistics. During the session, there were a lot of questions I was unable to answer because we ran out of time. Below are those questions and my responses (in italics). Many of these questions are answered by my various WintellectNOW videos. You can register for a free 14-day trial here.
1. Are memory leaks reflected in the used memory statistic of the task manager?
No, Task Manager doesn’t offer the best column for this. Use PerfMon.exe and watch a Process’ Virtual Bytes.
2. As a Windows OS advocate, I am curious on how Threading is implemented in Unix/Linux.
I answered this question on the call.
3. Can a thread ever run (or be set to run) for longer than one quantum?
Can you increase the time quantum for long running tasks
Not directly. You can raise a thread’s priority so it prevents lower-priority threads from running.
4. Can you point out the difference between threads and tasks?
A Task queues an operation to the thread pool. The thread pool then has one of its threads perform the operation. The thread pool threads are re-used over and over again to process all the queued operations. This reduces memory consumption and improves performance.
5. Could you elaborate more on the logical vs. real CPUs?
A physical CPU can perform one operation at a time. But sometimes, the physical CPU must pause and wait for RAM to complete some work. This causes the CPU to sit idle. Hyper-threaded CPUs can execute another thread during these pause times to improve overall system performance.
6. File Open Dialog was a common windows UI Control which exhibits this leak. Are there Windows APIs that tend to "leak" as well?
When you take a dependency on any technology (Microsoft or non-MS), you inherit its performance and efficiency problems. But, you saved yourself some time and energy. As a software developer you are charged with considering this tradeoff and determining if it is worth it for your application and your customers. Also, note that performance and efficiency are moving targets; that is, they change over time with later versions of the technology. With later versions things can get better or worse. So, when you take a dependency on some technology, these are the things you must be thinking about.
7. Given the advantages of using the thread-pool wouldn't it make sense to not allow the developer to create explicit threads and only provide access to "tasks" via the Win API?
Yes, in fact, the Windows Runtime (WinRT) API does not offer any functions allowing you to create threads; you MUST use the thread pool.
8. How are threads from background processes scheduled?
I’m not sure how you exactly define a “background process.” But, for the most part Windows schedules threads in a round-robin fashion without regard to which process the threads are in.
9. How could I make OS to schedule the most of CPU time working for an application?
This is dangerous thing to do and is discouraged. If the app goes into an infinite loop, then the rest of the system suffers greatly. However, you can raise the priority of threads in a process.
10. How does a thread pool help and how many threads should a pool have?
Thread pools help because they create threads and re-use them over and over again. This saves time because they do not constantly create and destroy threads. In addition, the thread pool knows how many CPUs the PC has and tries to create 1 thread per core to reduce context switching; this also improves performance.
11. How is the new Task class implemented? I had heard it was lighter weight than the Thread.
A Task is a small object in memory that knows how to queue a callback method to the thread pool. A task has no threads of its own. The task can monitor the lifetime of the queued item: did it complete? Did it thrown, did it return a value, etc.
12. How to interpret the benefit of parallel computing (increased performance) related to this thread waste of resource?
Most PCs can easily handle the allocation of a few MBs of memory in order for you app to take advantage of parallel processing. If you just strive for no more than 1 CPU per logical core, then memory consumption will stay low and performance will stay high.
13. If a quantum is 30 ms - how long does the context switch take?
How much time does it take the OS to do a context switch (i.e. what is context switch overhead relative to the size of a quantum)?
The time for a context switch various based on many factors: CPU speed, CPU architecture, and so on. But, what makes the performance even worse is that the CPUs cache is usually invalid after a context switch causing a lot of cache misses when accessing RAM.
14. If it is beneficial to create less thread as possible then what strategy should we choose when creating a WPF responsive Application? Any pattern or approach?
Typically UI apps (like WPF) have 1 GUI thread that process all user-interface events. Then, you queue up computationally-intensive work to the thread pool (via a Task) allowing the UI thread to respond to user input.
15. Jeff, even today we see some situations where we are not able to bring up task manager when the system is extremely busy.. why is this happening
It’s hard for to know for sure without being in front of the machine. But, my guess is that there may be some high-priority threads that are preventing Task Manager from displaying. This can sometimes happen with a bad device driver too.
16. Question set about duration of quantum, which you've indicated is 30ms - Has it always been 30 ms? What governs this duration? The HAL/clock interval?
Yes, the PC’s clock interval. There is a Win32 function that returns this info: GetSystemTimeAdjustment. Look at the lpTimeIncrement return value.
17. So are threads automatically recycled after a certain period of not being used?
If the thread are thread pol threads, then yes. If they are not thread pol threads, then no.
18. Threading topics apply to Azure programming?
Yes. Azure just creates virtual machines with Windows or other operating systems running in them. So information about threads apply to these VMs as well.
19. ThreadPool.GetAvailableThreads() show the answer as 1024 instead of 8. How is that possible when the number of cores in my PC are just 8?
In the remarks section for this method, it says that it returns the “number of additional worker threads that can be started.” This says that the thread can create this menay threads but not that it has actually created them.
20. What happens if you open another File Save As? Will more threads be orphaned?
No, it reuses the threads it created previously.
21. What is difference between Foreground and Background thread.
The .NET CLR kills a process as soon as all its foreground threads have stopped running (instantly destroying any background threads). So, foreground threads keep a process running while background threads do not.
22. What is fiber support in case of threading
Fibers a light-weight threads with the OS kernel doesn’t know anything about. The developer must write code to “context switch” from one fiber to another. Each fiber does have its own user mode stack but all fibers (on the same thread) share a kernel mode stack. .NET doesn’t support fibers and probably never will.
23. What is the performance effect of having multiple CPUs with multiple cores vs a single CPU with an equivalent number of cores?
For the most part, the perf would be the same. Sometimes, CPUs have to communicate with each other (like when taking a thread synchronization lock) and this communication is faster if the CPUs don’t have to talk through the bus on the motherboard.
24. How does async/await relate to threading?
These C# and VB language features allow you to perform I/O operations without blocking threads. This reduces the number of threads an application/service needs decreasing resource consumption and improving performance. I explain the value of all this in the video available here.
25. Is it always 30ms? Even in Windows 8.1?
On all versions of Windows to date, the timer interrupt fires at the same rate.
26. How do we get the additional threads started by File Open Dialogs (and other such controls) returned back to Thread Pool ?
You can’t control what a component does.
27. So those logical processors have their own CPU cache too? Otherwise, won't that impact performance?
The logical processors typically share the CPU’s cache. This is usually good as it allows bytes to be read once into the cache and shared by the other logical processors.
28. Is it wasteful to switch contexts if all the threads on the system are waiting most of the time?
No. But, if a thread does not want to wait, then allowing it to run without context switching is faster than introducing context switching.
29. Why is 32-bit arm about 1/2 of 32-bit x86 for overhead for memory for thread kernel object? Less data? More packing?
ARM CPUs have fewer CPU registers.
30. My question was: will there ever be such a concept supported by the .NET runtime, like the BEAM (Erlang virtual machine) threads, which said to be far more efficient and less resource expensive than threads, quicker context switch.
I’m not familiar with BEAM threads and what this actually means. I assume that they are like fibers and it is very unlikely that .NET will ever support fibers.
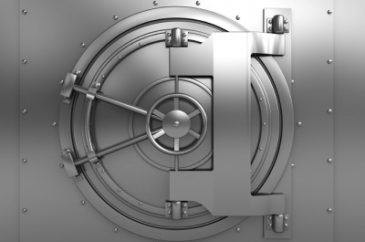
A Tale of Two Certies
For the past several months, Wintellect has been re-architecting the http://WintellectNOW.com website. We built a new storage model that separates users from accounts. This allows us to accommodate our corporate and enterprise customers better. For example, with the new system, an account can have multiple administrators allowing multiple people within a company to control who can and can’t watch videos. We’ve also been working on a much improved user experience so watching and learning from all our videos is much cleaner and faster. We haven’t launched these improvements publicly yet; but hope to in the next few months.
However, on August 23rd 2014, we did launch the new site internally for testing. Since we require SSL across the whole site, we needed a certificate and since the site will ultimately be deployed at http://WintellectNOW.com, we decided we’d use that certificate. WintellectNOW runs as a Microsoft Azure Cloud Service and so we went to our certificate authority’s website and re-keyed our WintellectNOW SSL certificate and uploaded it to our testing site: so far, so good.
A few days later, some customers contacted us telling us that the public WintellectNOW website was unreachable. Their browsers were reporting to us that there was something wrong with our certificate. Once we got the reports, we tried accessing the site from several devices. All mobile phones could access the site perfectly while browsers running on desktop computers reported certificate errors. Some of those browsers, such as Internet Explorer, refused access to the site, but others, like Safari, let us continue to the site after reporting the certificate error. Even more interesting is that some browsers just said there was a problem with the certificate. The failure inconsistencies and the fact that we didn’t touch the public-facing site made the whole thing quite puzzling.
It took us about 30 minutes (with some more experimentation) to reason it out. Our certificate authority revokes a certificate when you re-key it. Apparently, not all certificate authorities do this but ours does. And, not all browsers honor this, but some do. I would have expected a “NOTE: Re-keying a certificate revokes your current certificate.” in big bold red letters to be on the CA’s webpage where you do the re-keying but no such text is there. Now that we knew what was wrong, it was really easy to fix it: we uploaded the new certificate to the public WintellectNOW website and then uploaded a new Azure Service Configuration (.cscfg) file to our role instances and the site was back again working for everyone within 5 minutes.
While this was a fire-drill that had several Wintellectuals working on the problem, it was a great lesson to learn (which is why I wanted to share it with this blog post). And, it was also reassuring to see how well we handled an unexpected problem as a team and how quickly we were able to resolve the issues for the customers who were experiencing problems.
We are very excited about the WintellectNOW website as is stands today and about our plans for its future. If you’d like to explore our deep, rich technical contact for two weeks free, register using this link: https://www.wintellectnow.com/Account/Promo/JeffreyR-2013.
On a side note: We license and optionally manage the WintellectNOW website to other companies who use it as their own video delivery platform. The architectural changes we’ve been making to the site helps these companies too. Please contact Wintellect if you’d like to use the WintellectNOW website for you own company’s video delivery system.